PHP int, or integer, is a data type that represents whole numbers, such as 1, 100, or -5, without any decimal places. We can use integers in PHP for mathematical operations and for comparing numbers in code.
The term ‘int’ just means integer in PHP, and the two words are permitted to be utilized.
Moreover, there are some constants in PHP that set the limits for integers. These limits include the maximum and minimum values an integer can have.
PHP_INT_SIZE
keeps track of how many bytes something uses. Simply put, it’s usually 8 bytes.
PHP_INT_MAX
gives you the largest possible integer value in PHP. It’s a big number: 9223372036854775807.
-
PHP_INT_MIN
shows the smallest whole number in PHP, and it’s – 9223372036854775808.
Additionally, remember that the size of a number in PHP depends on the platform you’re using. But generally, it allows for a maximum value of 2 billion to be stored in an integer variable.
Anyway, PHP has 4 integer (int) types, which are:
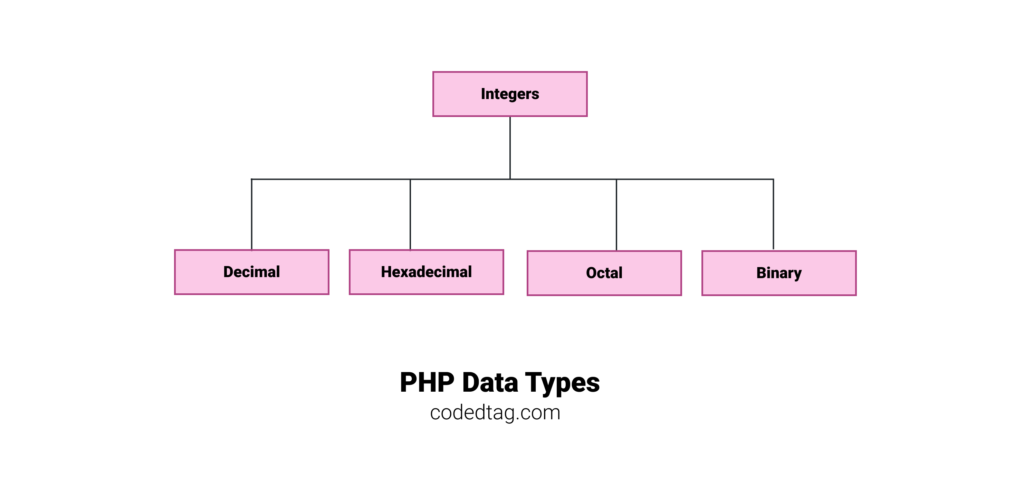
Let’s focus on each one in-depth.
Exploring Decimal Numbers in PHP
Basically, numbers with a decimal point are known as decimal numbers. This point, which is usually on the right side of the number, may have one or more digits. Ten is the maximum number of digits in base 10 that come after the decimal point.
To make numbers in PHP simpler to understand, group them together with underscores. For example:
<?php
echo 4_487_456 // 4487456 decimal;
echo 145 // 145 decimal;
?>
Anyway, let’s go on to the next section, where we’ll look at hexadecimal numerals in more depth.
Understanding Hexadecimal Numbers
Typically, hexadecimal refers to base-16 digits between 0 and 15. It deals with single-digit integers ranging from 0 to 9. The extra six digits are replaced with characters in the sequence of ‘a’ to ‘f’ based on their individual records.
The following list shows the double digits that would be used in place of the alphabetical characters:
- 10 -> a
- 11 -> b
- 12 -> c
- 13 -> d
- 14 -> e
- 15 -> f
Hexadecimal numerals represent values beyond single-digit integers using the letters A through F. Understanding the difference between ordinary numbers (0–9) and letters (A–F) is crucial to understanding the system.
To combine letters and numbers, start at the beginning with ‘0x’. For a clear example, see below:
<?php echo 0x2FD; // decimal 765 ?>
The result in this model is 765. If you go back and read this part again, you will see that we are using base-16 for the hexadecimal scheme. This implies that the calculation will look like the picture below if we want to convert a number from hexadecimal to digits.

Explaining the Calculation Process
Check the three numbers with the red circle, from right to left: 0, 1, and 2. These numbers begin with zero and address the kind. For instance, a 162 type denotes 16 x 16, while a 164 type denotes 16 x 16 x 16 x 16, and so on.
Let’s break down the figure line by line, starting from the left.
- Hexadecimal is represented by adding “0x” to the beginning of numerals.
- As discussed in the last section, you should enter the numbers in the resultant line, starting with zero and going from right to left.
- Increase the primary number to (2 x 16)₂ from the left, and it will increase to (2 x (16 x 16). The result is 512.
- The next number expands to (15 x (16)): (15 x 16) ₁. The result is 240.
- Then, there is a similar growth in the number (13 x 16) 0. The result is 13.
- Adding up these red font results gives us the number 765.
You now have a solid understanding of the foundations of working out when dealing with additional PHP number (int) types.
Anyway, let’s move on to octal numbers in PHP.
Octal Numbers in PHP
There is a digit range for octal numbers between (0) and (7), encompassing eight numbers in total (base 8). To define octal numbers, simply add ‘0’ or (‘0o’ for PHP 8.1.0) at the beginning. For example:
<?php
echo 0457; // decimal 303
?>
The figure below provides an explanation and illustrates how to calculate it.
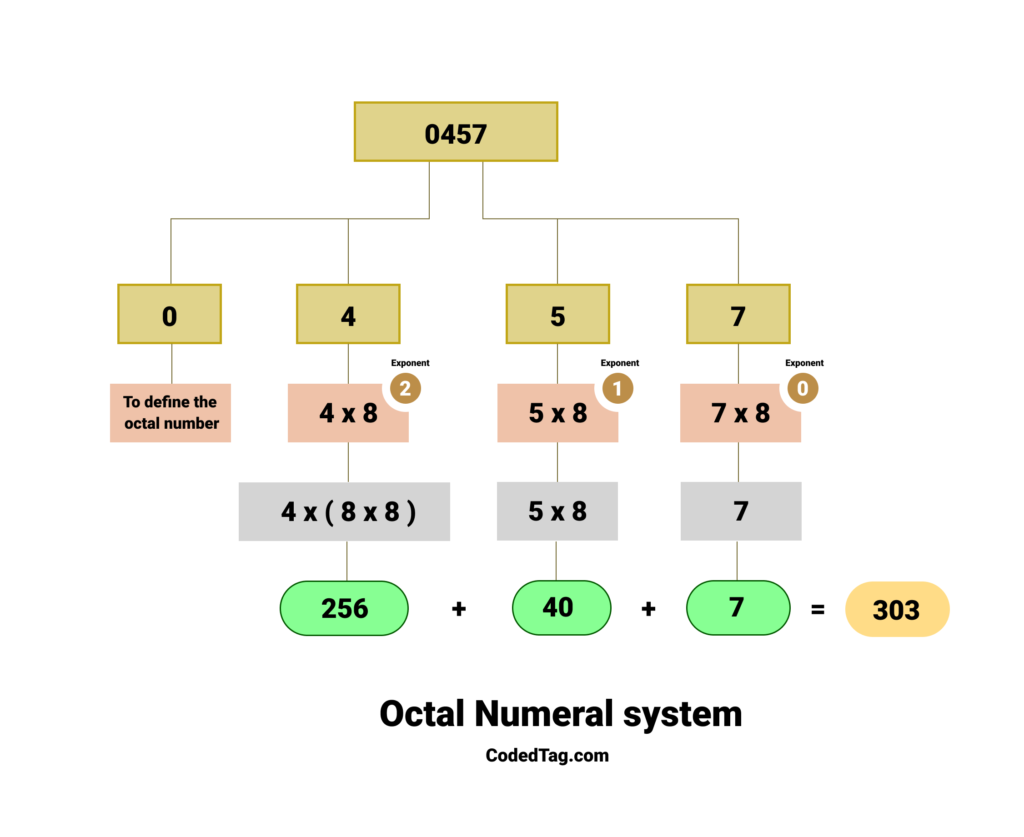
Explaining the Calculation Process
The number (0457) is an octal representation. In the octal system, the digits extend from (0) to (7). The equivalent decimal value is as follows:
4 * (8^2) + 5 * (8^1) + 7 * (8^0) = 303
Therefore, it will output (303) in decimal notation.
Let’s delve deeper into another type of PHP integer—binary numbers.
Understanding Binary Numbers
The binary number system just uses the (0s) and (1s), which are called binary bits. This system starts with 0s.
Additionally, binary numbers are very important to machine code, which is a basic type of computer language.
In this section, you will cover the number systems and how they would be calculated. Here is an example.
<?php echo 0b101111; // Decimal 47 ?>
The figure below shows how to calculate the binary numeral system.

So, look at this binary number (0b101111). It starts with the prefix (0b), which indicates that the following digits are in binary notation.
If you focus on the following digits of this prefix (0b), which is (101111), you will see it shows a series of powers of (2), like the one below.
(1) x ((2 ^ 0) = ( 1 ) = (1)) = 1
(1) x ((2 ^ 1) = ( 2 ) = (2)) = 2
(1) x ((2 ^ 2) = ( 2 x 2 ) = (4)) = 4
(1) x ((2 ^ 3) = ( 2 x 2 x 2) = (8)) = 8
(0) x ((2 ^ 4) = ( 2 x 2 x 2 x 2 ) = (16)) = 0
(1) x ((2 ^ 5) = ( 2 x 2 x 2 x 2 x 2) = (32)) = 32
Here is an analysis of the above calculation.
- The rightmost digit is (1), which represents (2 ^ 0), so usually the first digit in the right position is (1).
- In the next number, the digit is (1), so it exposes the exponentiation like this: (2 ^ 1) = (2).
- We are still moving from right to left in the calculation. The next digit is (1), which is the number (2) that equals (2 ^ 2) = (4).
- And then the following number is (1), which would take (2 ^ 3) = (8).
- The next digit is 0, representing (2^4). Since this digit is (0), it contributes (0) to the total.
- In the final digit, which is the most left number, it is (1). It takes (2^5), and that equals (32).
Let’s get the sum of the whole calculated number:
32 + 0 + 8 + 4 + 2 + 1 = 47
So, (0b101111
) in binary is equal to (47
) in decimal.
Anyway, let’s summarize it.
Wrapping UP
In this tutorial, you learned what PHP (int) integers are, including their types such as decimal, hexadecimal, octal, and binary. We started with an explanation of the basics of each type, with examples that exposed some techniques for calculations.
So, in the first section, we discuss PHP_INT_SIZE, PHP_INT_MAX, and PHP_INT_MIN constants. These help you understand the size limits of PHP numbers.
And then you understood the decimal numbers and how they work in PHP.
In the following section, we explored the hexadecimal numbers, and you learned what they look like and how to convert them to decimals.
Then, we explained what octal numbers are and how to use them in PHP with real examples.
In this tutorial, you also got an understanding of what binary numbers are and learned about their importance in computer computing.
Thank you for reading. Happy Coding!