The PHP function parameters are data or variables that can be passed through the function. In the other hand, the PHP function arguments are the values that can be assigned when call the function.
Table of Contents
Before getting started, you have to understand the difference between parameters and arguments.
The Difference between the Arguments and the Parameters in the Functions
The parameters and arguments are parts in PHP function and two both working together. So if the function has no parameters or has a parameter with default value. That potentially means there are no arguments.
The following figure shows you an example.
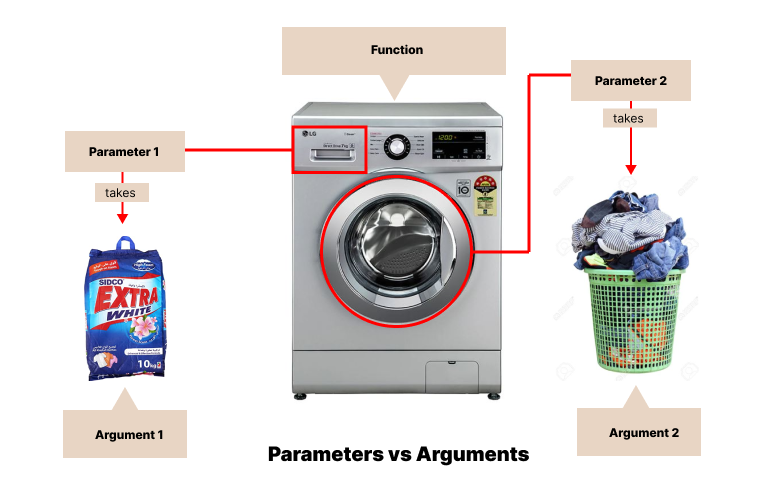
So the arguments are values and the parameters are fields of the function which accepts the values from the top. And then it would be executed as the function part.
All the elements are working together in the function to giving us the final result. So it may print the result or returning it.
How to Create Parameters and Arguments within the Function in PHP
The parameters would be placed inside the braces of functions (...)
which the input of the function, that can accept all coming values from the calling places.
The basic syntax of parameters would be as the below example.
<?php
function codedtag_function( $param1, $param2, $param3, $param4 ) {
// ==> Code Statements
}
?>
While, the arguments are the values that can be assigned when you call the function from another place in the PHP script.
The following example shows you how it does work.
<?php
codedtag_function( "Value 1", "Value 2", "Value 3", "Value 4" );
?>
Sometimes, you do a mistake when you set an argument instead of another one, that will make an issue in the inputs of function.
So, you have to do a comment beside each argument to expose a brief for the current value in each argument, for example.
<?php
codedtag_function(
"Value 2", // Value 1
"Value 1", // Value 2
"Value 4", // Value 3
"Value 3" // Value 4
);
?>
PHP Default Parameters
The default parameters are optional in the PHP functions which define a default value when you create a function. So when you call the function you will have an option to pass this value or not.
For example.
<?php
function is_admin( $is_admin = 0 ) {
$admin = 1;
if ( $admin == $is_admin ) {
return true;
}
return false;
}
var_dump( is_admin() ); // bool(false)
var_dump( is_admin(1) ); // bool(true)
?>
So, the default parameter already assigned with 0 and you have an option to insert this argument when you call the function if this user is_admin so it will return false value by default.
Note that, when you assign a default parameter you should make it in the last order. So if you have 3 parameters and one of them has a default value, you have to put it at the last position. For example.
<?php
// => This has a default value with wrong position
function is_admin( $param1 = " ", $param2, $param3 ) { .. }
// Correct position for default value
function is_admin( $param2, $param3, $param1 = " " ) { .. }
?>
Passing Function Arguments by Reference
As I mentioned before the function arguments are the values that can be assigned when you call function in another place.
For example.
<?php
function codedtag_refs( &$welcome ) {
$welcome .= " Peter";
}
$welcome = "Hello";
codedtag_refs($welcome);
print( $welcome ); // Hello Peter
?>
Another example.
<?php
$number = 1;
function increase_number( &$add_number ) {
$add_number += 22;
}
increase_number($number);
echo $number; // 23
?>
Also you can assign values by global variable, that means you will able to change the value of the variable from the function by using global statement.
<?php
$counter = 10;
function add_six( $new ) {
global $counter;
$counter += $new;
}
add_six(20);
echo $counter; // 30
?>
Passing Function Arguments by Value
This way will not effect in the value of global variable, let’s take an example.
<?php
function add_number( $new ) {
$new += 5;
}
$counter = 10;
add_number($counter);
echo $counter; // 10
?>
Wrapping Up
In this article, you understood what are parameters, arguments and how they work in PHP functions.