Bitwise operators in PHP enable programmers to perform operations at the binary level, making it possible to manipulate binary digits (bits) directly.
These operators can be employed for tasks such as setting or clearing specific bits, checking the status of individual bits, and performing various bitwise operations like AND, OR, XOR, left shift, and right shift.
Before we dive in, let’s familiarize ourselves with some details about binary representation.
Understanding Binary Representation
Binary representation is the fundamental system computers use to store and process data. In the binary system, digits, or bits, can only take on two values: 0 or 1. These bits are the building blocks of binary numbers, where each position represents a power of 2. For instance, the rightmost bit corresponds to 2^0, the next to 2^1, and so on.
To convert binary to decimal, you add up the decimal values of each bit based on its position. For example, the binary number 101010 translates to
- 25×1=3225×1=32
- 24×0=024×0=0
- 23×1=823×1=8
- 22×0=022×0=0
- 21×1=221×1=2
- 20×0=020×0=0
Adding these values together (32+0+8+0+2+032+0+8+0+2+0), we get the decimal number 42.
To better understand, please refer to the figure below.
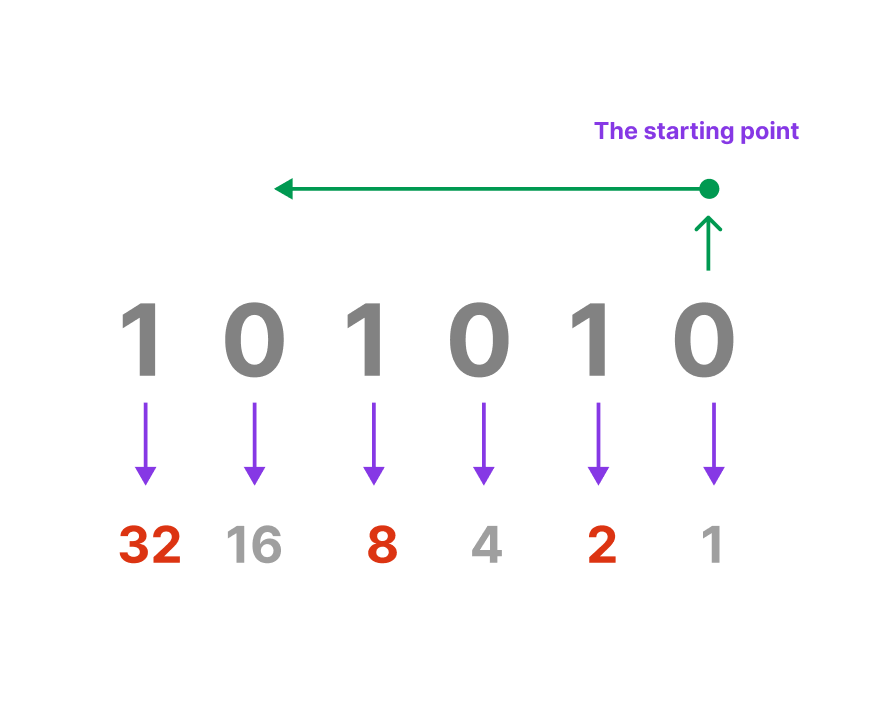
In computing, binary is extensively used for memory storage. Each bit in a binary number can represent the state of a memory cell, either 0 or 1. This binary representation is also the foundation for interpreting digital signals in computers and communication systems. In digital electronics, signals are often categorized as low voltage (0) or high voltage (1).
One practical application of binary representation is in bitwise operations, where individual bits in binary numbers are manipulated. Let’s delve into these operators in the following sections.
Bitwise AND Operator (&)
The bitwise AND operator (&) in PHP is used to perform a bitwise AND operation on each bit of two integers. It compares each bit of the first operand with the corresponding bit of the second operand, and the result is 1 only if both bits are 1. If either or both of the bits are 0, the result is 0.
Let’s take an example using two integers, a= 55 (Binary: 110111) and b = 100 (Binary: 1100100)
<?php
$a = 55; // Binary: 110111
$b = 100; // Binary: 1100100
$result = $a & $b;
?>
PHPHere’s the bitwise AND operation for each bit:
110111 (Binary representation of $a)
& 1100100 (Binary representation of $b)
-------------------------------------------------------------
1100100 (Result of $a & $b, equivalent to decimal 36)
PlaintextSo, $a equals 55 which is “110111”, as calculated from the binary representation shown in the figure below.

Similarly, you need to obtain the binary representation of $b for the final calculation, as illustrated in the following figure.
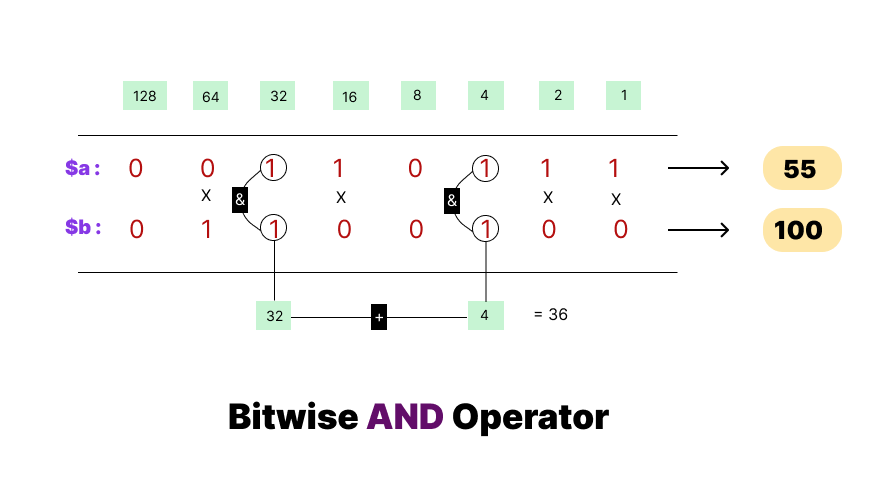
In this example, the binary representation of a is 1101112 and b is 11001002. The bitwise AND operation compares each pair of corresponding bits: 1 & 1 1&1 results in 1 1, 1 & 1 1&1 results in 1 1, 0 & 0 0&0 results in 0 0, 1 & 0 1&0 results in 0 0, 1 & 1 1&1 results in 1 1, and 1 & 0 1&0 results in 0 0. Therefore, the final result of a & b is 11001002, which is equivalent to decimal 36.
Anyway, in the following section, I will discuss the bitwise OR operator. Let’s move forward.
Bitwise OR Operator (|)
The Bitwise OR operator (|
) is a fundamental operator used in programming languages to perform bitwise OR operations on the individual bits of two integers. In PHP, the Bitwise OR operator is represented by the pipe symbol (|
). When the Bitwise OR operator is applied to two integers, it compares the corresponding bits of the binary representations of those integers and sets the result bit to 1 If any of the corresponding bits in the operands is set to 1.
For an example:
<?php
$number_1 = 55;
$number_2 = 100;
// The Bitwise OR operator is represented by |
$result = $number_1 | $number_2;
// Output the result
echo $result; // => Output: 119
?>
PHPIn this example, we have two integers, $number_1
with a decimal value of 55 and $number_2
with a decimal value of 100. When we apply the Bitwise OR operator (|
) to these numbers, the binary representations of each number are considered at the bit level.

Another explanation for it.
0110111 (Binary representation of $number_1)
| 1100100 (Binary representation of $number_2)
---------------------------------------------------
1110111 (Result of $number_1 | $number_2, equivalent to decimal 119)
PlaintextThe binary representation of 55 is 110111
, and the binary representation of 100 is 1100100
. Performing the Bitwise OR operation on each corresponding pair of bits, we get the binary result 1110111
, which is equivalent to the decimal value 119.
So, when we echo $result
, the output will be 119
, demonstrating the result of applying the Bitwise OR operator to $number_1
and $number_2
.
Let’s proceed to the next section to understand how to extract binaries using the XOR operator.
Bitwise XOR Operator (^)
The Bitwise XOR operator (^) compares each bit of two numbers and returns 1 where the bits are different and 0 where they are the same. In other words, it performs the exclusive OR operation on individual bits.
Let’s see an example:
<?php
$number_1 = 55; // Binary: 00110111
$number_2 = 100; // Binary: 1100100
// Bitwise XOR operation
$result = $number_1 ^ $number_2; // Binary result: 1111111
// Convert the binary result back to decimal
echo bindec($result); // Output: 83
?>
PHPHere’s a step-by-step explanation:

$number_1
in binary is00110111
.$number_2
in binary is1100100
.- The Bitwise XOR operation is performed on each corresponding pair of bits. When the bits are identical, the outcome is 0; if they differ, the result is 1.
- The binary result of the XOR operation is
1111111
. - So, the output of
echo $number_1 ^ $number_2;
is83
.
For another explanation:
00110111 (Binary representation of $number_1)
^ 01100100 (Binary representation of $number_2)
---------------------------------------------------
01010011 (Result of $number_1 ^ $number_2, equivalent to binary)
PlaintextLet’s explore another type of bitwise operator in the following section, specifically the bitwise NOT operator.
Bitwise NOT Operator (~)
The Bitwise NOT operator (~
) is a unary operator that is used to perform bitwise negation on the individual bits of an integer. In PHP, the Bitwise NOT operator is represented by the tilde symbol (~
). When the Bitwise NOT operator is applied to an integer, it flips each bit of the binary representation of that integer, changing 0s to 1s and 1s to 0s.
Let’s consider an example in PHP:
<?php
$number = 55;
// The Bitwise NOT operator is represented by ~
$result = ~$number;
// Output the result
echo $result; // => Output: -56
?>
PHPIn this example, we have an integer $number
with a decimal value of 55. When we apply the Bitwise NOT operator (~
) to this number, the binary representation of the number is considered, and each bit is flipped.
The binary representation of 55 is 110111
. Applying the Bitwise NOT operation to each bit, we get the binary result 111000
, which is equivalent to the decimal value -56 in two’s complement form.
So, when we echo $result
, the output will be -56
, demonstrating the result of applying the Bitwise NOT operator to $number
.
Let’s move on to discussing the bitwise left shift operator in the following section.
Bitwise Left Shift Operator (<<)
The Bitwise Left Shift operator (<<
) is a binary operator used in programming languages to shift the bits of an integer to the left by a specified number of positions. In PHP, the Bitwise Left Shift operator is represented by the double less-than symbol (<<
). When the Bitwise Left Shift operator is applied, each bit in the binary representation of the operand is shifted to the left by a certain number of positions, and zeros are filled in on the right.
For example:
<?php
$push = 4;
$number = 10;
// The Bitwise Left Shift operator is represented by <<
$result = $number << $push;
// Output the result
echo $result; // => Output: 160
?>
PHPIn this example, we have a variable $number
with a decimal value of 10, and a variable $push
with a value of 4. When we apply the Bitwise Left Shift operator (<<
) to $number
by $push
positions, each bit in the binary representation of $number
is shifted four positions to the left, and zeros are filled in on the right.

The binary representation of 10 is 1010
. Shifting it to the left by 4 positions, we get the binary result 1010000
, which is equivalent to the decimal value 160.
Next, we’ll delve into the details of the bitwise right shift operator in the upcoming section.
Bitwise Right Shift Operator (>>)
The Bitwise Right Shift operator (>>
) is a binary operator used in programming languages to shift the bits of an integer to the right by a specified number of positions. In PHP, the Bitwise Right Shift operator is represented by the double greater-than symbol (>>
). When the Bitwise Right Shift operator is applied, each bit in the binary representation of the operand is shifted to the right by a certain number of positions, and zeros are filled in on the left.
Let’s see an example:
<?php
$pull = 2;
$number = 10;
// The Bitwise Right Shift operator is represented by >>
$result = $number >> $pull;
// Output the result
echo $result; // => Output: 2
?>
PHPIn this example, we have a variable $number
with a decimal value of 10, and a variable $pull
with a value of 2. When we apply the Bitwise Right Shift operator (>>
) to $number
by $pull
positions, each bit in the binary representation of $number
is shifted two positions to the right, and zeros are filled in on the left.
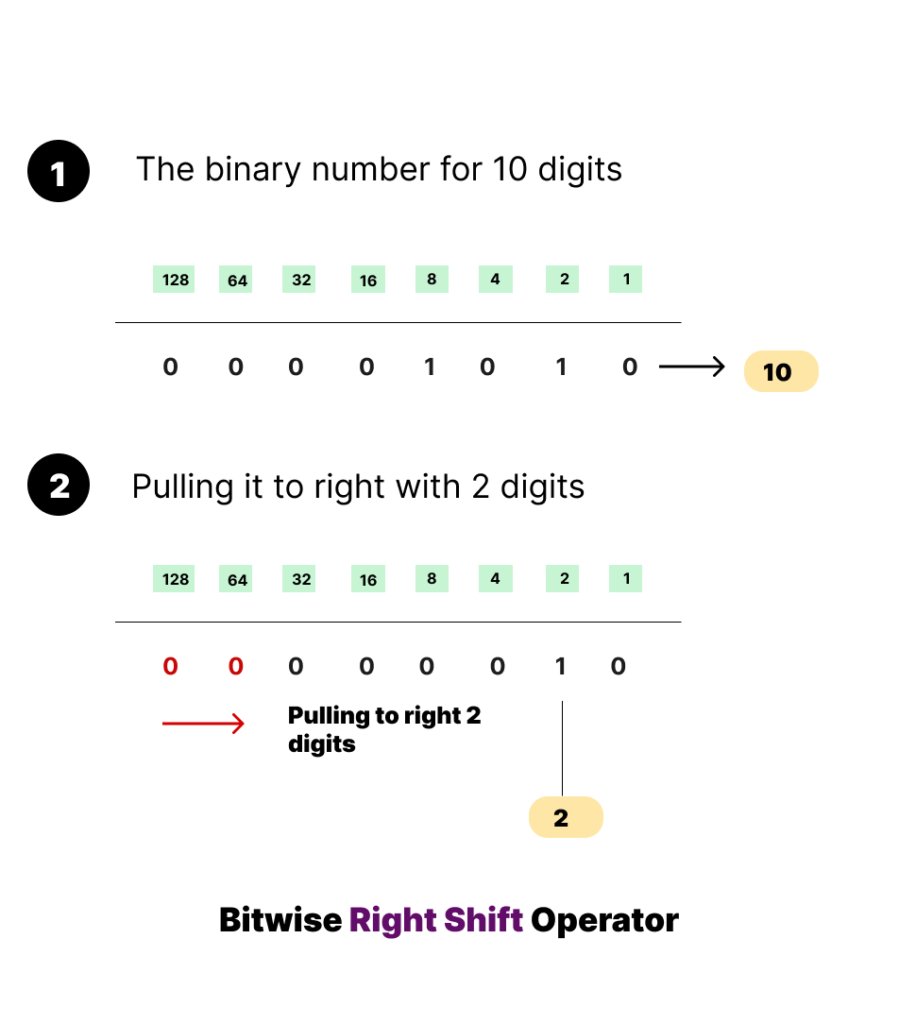
The binary representation of 10 is 1010. Consequently, shifting it to the right by 2 positions yields the binary result 10, which is equivalent to the decimal value 2.
At this point, we have acquired an understanding of the bitwise operator system, but we may be unsure about its practical applications in PHP and the reasons for employing it. In the section below, we will delve into the usage of a common example to illustrate the practical applications of bitwise operators.
Using Bitwise Operators for Flags and Permissions
PHP Bitwise operators are often used in programming to work with flags and permissions efficiently. Flags and permissions are commonly represented using individual bits in an integer, allowing multiple options or settings to be stored compactly within a single variable. This is particularly useful when working with limited resources or when optimizing storage.
Let’s discuss how PHP bitwise operators can be used for flags and permissions with a simple example:
<?php
// Define constants for permissions
const READ = 1; // Binary: 0001
const WRITE = 2; // Binary: 0010
const EXECUTE = 4; // Binary: 0100
// Set initial permissions
$userPermissions = 0;
// Grant permissions using bitwise OR operator (|)
$userPermissions = $userPermissions | READ | WRITE;
// Check if a specific permission is granted using bitwise AND operator (&)
$hasReadPermission = $userPermissions & READ;
$hasWritePermission = $userPermissions & WRITE;
$hasExecutePermission = $userPermissions & EXECUTE;
// Display results
echo "User Permissions: $userPermissions\n";
echo "Has Read Permission: " . ($hasReadPermission ? 'Yes' : 'No') . "\n";
echo "Has Write Permission: " . ($hasWritePermission ? 'Yes' : 'No') . "\n";
echo "Has Execute Permission: " . ($hasExecutePermission ? 'Yes' : 'No') . "\n";
?>
PHPIn this example, we employ three constants (READ, WRITE, and EXECUTE) to represent different permissions. Each constant has a unique binary value with a single bit set to 1. Subsequently, we utilize a variable $userPermissions to store the combined permissions.
When it comes to granting permissions, we employ the bitwise OR operator (|) for the process. For instance, $userPermissions = $userPermissions | READ | WRITE; effectively grants both read and write permissions.
Moving on to the checking of permissions, the bitwise AND operator (&) is employed to determine if a specific permission is granted. If the result is non-zero, the permission is indeed granted. For instance, $hasReadPermission = $userPermissions & READ; checks if the read permission is granted.
Let’s summarize it.
Wrapping Up
bitwise operators in PHP provide a powerful means for manipulating binary data directly, enabling programmers to perform operations at the binary level. These operators offer functionalities such as setting or clearing specific bits, checking bit statuses, and executing various bitwise operations like AND, OR, XOR, left shift, and right shift.
Before delving into PHP bitwise operators, understanding binary representation is crucial. The binary system, based on bits that can only be 0 or 1, forms the foundation of computer data storage and processing. Binary representation plays a vital role in tasks like memory storage, digital signal interpretation, and bitwise operations.
The bitwise AND operator (&) compares corresponding bits of two integers, resulting in 1 only if both bits are 1. Meanwhile, the bitwise OR operator (|) sets the result bit to 1 if at least one of the corresponding bits is 1.
Additionally, the bitwise XOR operator (^) returns 1 where bits are different and 0 where they are the same. Moving on to the bitwise NOT operator (~), it performs bitwise negation, flipping each bit of an integer. Finally, the bitwise left shift operator (<<) shifts bits to the left, and the right shift operator (>>) shifts bits to the right.
These operators find applications in various programming scenarios, providing efficient tools for tasks involving binary manipulation. As we continue exploring the intricacies of programming, the understanding and utilization of PHP bitwise operators remain valuable skills.
Thank you for reading. For more tutorials in PHP, please visit this page.
For further reference and detailed information on PHP functions and syntax, you can explore the official PHP manual available at PHP Manual.