PHP has a number of predefined constants that give you some information about the language and its environment. You can call them through your PHP script code.
Table of Contents
These constants are specific values that don’t change and are just designed to be called during the code execution process.
PHP itself includes something called the Zend Engine and several other modules that help communicate with web servers; its name is SAPI modules.
We can categorize them into groups based on their functionalities and purposes; let’s begin with that.
PHP Version Information
These constants are used to retrieve information about the current PHP version that is installed on your machine. The most common one is the PHP_VERSION constant. Let’s see an example of it:
if ( version_compare(PHP_VERSION, '8.0.30') !== 0) {
echo "This version is not 8.0.30";
}
In the following paragraphs, you will see all constants of PHP Version Information with a brief explanation.
- PHP_VERSION to retrieve the current installed version of PHP.
- PHP_MAJOR_VERSION to capture the current major version number of the PHP interpreter.
- PHP_MINOR_VERSION to display the minor version of the PHP interpreter.
- PHP_RELEASE_VERSION to show the current release version of PHP.
- PHP_VERSION_ID to merge major, minor, and release versions into a numeric representation.
- PHP_EXTRA_VERSION includes additional version details, such as pre-release or patch information.
- PHP_ZTS checks if PHP functions properly in situations where multiple things are occurring at the same time.
- PHP_DEBUG tells us if PHP has a special feature for finding and fixing problems in our code.
The following image shows you all of these constants in one shot.
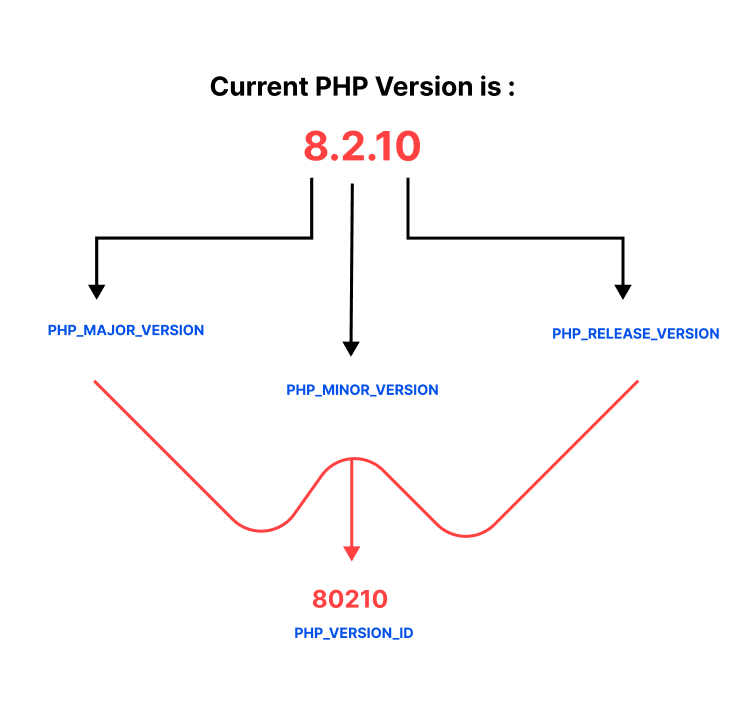
Anyway, let’s move to the predefined constants of system information in PHP
System Information
The constants here are used to get information about the operating system, which appears as an environment of the current PHP installer. Let’s see each one in details:
- PHP_OS: Represents the operating system PHP is running on.
- PHP_OS_FAMILY: Classifies the OS family, such as Windows, Unix, or macOS.
- PHP_SAPI: Identifies the Server API, indicating how PHP interacts with web servers.
- PHP_EOL: Represents the end-of-line sequence used in PHP scripts.
- PHP_INT_MAX: Maximum value for integers in PHP.
- PHP_INT_MIN: Minimum value for integers in PHP.
- PHP_INT_SIZE: Size of an integer in bytes.
- PHP_FLOAT_DIG: Decimal digits of precision for floating-point numbers.
- PHP_FLOAT_EPSILON: Smallest representable positive floating-point number.
- PHP_FLOAT_MIN: Minimum floating-point number representable.
- PHP_FLOAT_MAX: Maximum floating-point number representable.
- DEFAULT_INCLUDE_PATH: Default search paths for include files.
- PEAR_INSTALL_DIR: Directory where PEAR packages are installed.
- PEAR_EXTENSION_DIR: Directory for PEAR extensions.
- PHP_EXTENSION_DIR: Directory for PHP extensions.
- PHP_PREFIX: Common prefix for PHP installations.
- PHP_BINDIR: Directory containing PHP binary.
- PHP_BINARY: Path to the PHP binary.
- PHP_MANDIR: Directory containing manual pages.
- PHP_LIBDIR: Directory for PHP libraries.
- PHP_DATADIR: Directory for read-only data used by PHP.
- PHP_SYSCONFDIR: Directory for system-wide configuration files.
- PHP_LOCALSTATEDIR: Directory for modifiable runtime data.
- PHP_CONFIG_FILE_PATH: Path to the PHP configuration file.
- PHP_CONFIG_FILE_SCAN_DIR: Directory for additional ini files.
- PHP_SHLIB_SUFFIX: Shared library suffix (e.g., “.so” on Unix).
- PHP_FD_SETSIZE: Maximum number of file descriptors in a set.
Let’s move into the following part, which comprises the error handling constants.
Error Handling
These constants are used to control how errors are reported and handled in your scripts. Let’s see them in the list below.
- E_ERROR to E_USER_DEPRECATED: Numerical representations of PHP error levels, ranging from critical errors (E_ERROR) to user-triggered deprecations (E_USER_DEPRECATED).
- E_ALL: Represents all error levels, indicating that all types of errors should be reported.
- E_STRICT: A level emphasizing strict adherence to coding standards, promoting compatibility.
- COMPILER_HALT_OFFSET: Special offset value for
__halt_compiler()
, indicating the end of file for embedded data.
Let’s move into the section below, which covers Boolean and Null Values constants.
Boolean and Null Values
These are predefined words in the PHP core used to handle logical operations.
- true
- false
- null
Windows-specific Constants
PHP_WINDOWS_EVENT_CTRL_C (int): An integer constant in PHP representing the Ctrl+C (SIGINT) event on Windows. Used for handling interrupt signals, typically to gracefully terminate a process or handle user interruptions.
PHP_WINDOWS_EVENT_CTRL_BREAK (int): An integer constant in PHP representing the Ctrl+Break event on Windows. This is another signal event that can be used for special handling in a PHP script, allowing developers to respond to specific user inputs or system signals.
Let’s summarize it
Wrapping up
A set of predefined constants in PHP provides insights into the language and environment. Easily accessible in your code, these constants remain static, designed for various functionalities.
We explored PHP Version Information, System Information, and Error Handling, categorizing constants by their purposes. The journey led us through details of PHP’s interaction with web servers, operating system details, and error control mechanisms.
We delved into Boolean and Null Values and even explored Windows-specific constants. These constants serve as invaluable tools for developers, enhancing code adaptability and facilitating efficient problem-solving. As we navigate this dynamic landscape, PHP constants stand as pillars, providing stability and functionality to our coding endeavors.
Thank you for reading. Happy Coding!